Understanding Shell Sort Algorithm with C#
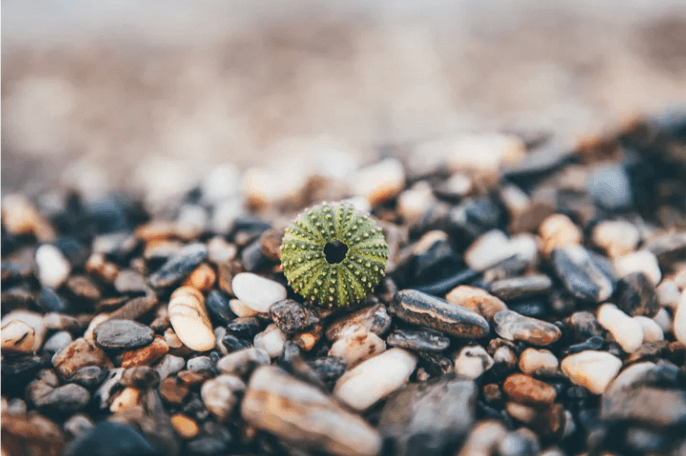
Photo by Nick Karvounis on Unsplash
The world of sorting algorithms is vast and intriguing. While some algorithms have grown popular due to their efficiency and performance, others have remained in the shadows despite their unique approaches. One such algorithm is the Shell Sort. In this article, we’ll dive deep into Shell Sort, its C# implementation, and understand its advantages and drawbacks.
A Brief Introduction to Shell Sort
Shell Sort, named after its inventor Donald Shell, is an interesting algorithm that generalizes the idea of insertion sort by allowing the exchange of elements that are far apart. But what sets it apart from a regular insertion sort?
The Mechanics of Shell Sort
While insertion sort compares and positions adjacent elements, Shell Sort ups the ante by comparing elements that are a specific ‘gap’ apart. This gap starts as a large value and gradually reduces, aiming to position distant elements in their correct positions early, making it easier to place closer elements later.
Imagine sorting a long line of dancers who only compare themselves with dancers a certain distance apart. As the song progresses, this distance reduces, and by the end of the song, everyone is comparing with their immediate neighbors (just like insertion sort). This ‘dancing’ technique ensures that by the time we reach regular insertion sort behavior, the list is nearly sorted, making the entire process faster.
The C# Dance: Implementing Shell Sort
Let’s see how this dance of elements can be translated into C#:
public static void ShellSort(int[] arr)
{
int n = arr.Length;
for (int gap = n / 2; gap > 0; gap /= 2)
{
for (int i = gap; i < n; i++)
{
int temp = arr[i];
int j;
for (j = i; j >= gap && arr[j - gap] > temp; j -= gap)
{
arr[j] = arr[j - gap];
}
arr[j] = temp;
}
}
}
The outer loop dictates the gap between comparisons. The inner loop is akin to insertion sort but with a twist — instead of comparing adjacent elements, it compares elements gap positions apart.
Why Opt for the Shell Sort?
- Adaptive Nature: If your list is partially sorted, Shell Sort could speed through it!
- Parallel Potential: Its design lets parts of it run concurrently, leveraging multi-core architectures.
- Memory Efficiency: Being an in-place sort, it doesn't demand additional memory.
The Other Side of the Coin: Drawbacks
- The Gap Dilemma: Its efficiency can hinge on the chosen gap sequence. Though many sequences have been proposed, the perfect one remains elusive.
- Stability Concerns: Shell Sort doesn’t guarantee the order of equal elements, making it unstable.
- Speed Limitations: While it shines with medium lists, larger ones are better sorted with algorithms like merge sort or quick sort.
Making the Choice: When to Use Shell Sort?
- If you’re dealing with a medium-sized list, especially one that’s potentially partially sorted.
- Memory constraints are tight.
But refrain when:
- Handling vast data sets.
- Stability in sorting is non-negotiable.
Wrapping Up
Shell Sort offers a unique blend of the simplicity of insertion sort with the promise of faster results. While it may not be the go-to algorithm for all scenarios, understanding its nuances is crucial for any programming aficionado.
References:
- Shell, Donald L. (1959). A High-Speed Sorting Procedure. Communications of the ACM.